Pandas
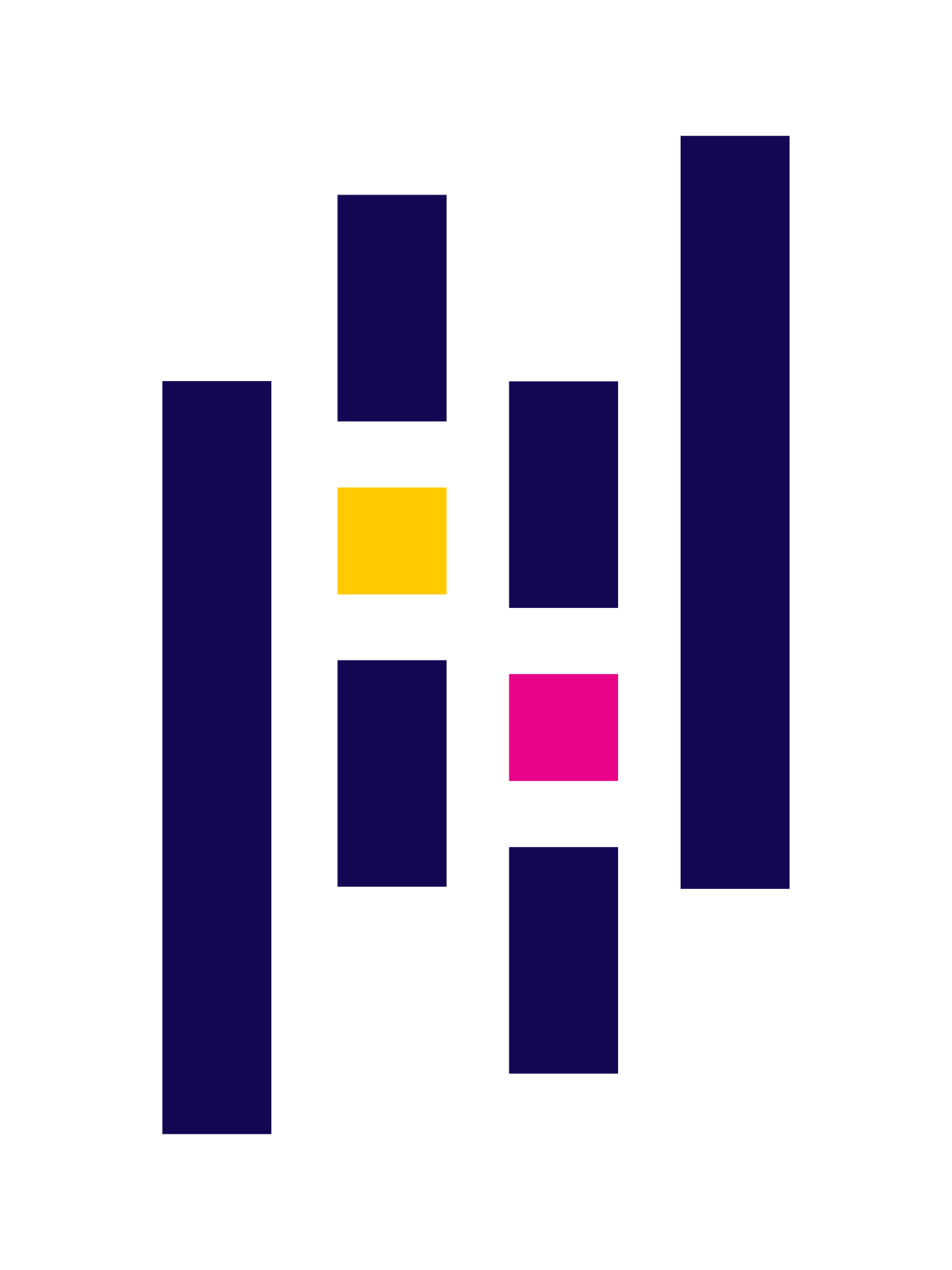
Plugin: python.d.plugin Module: pandas
Overview
Pandas is a de-facto standard in reading and processing most types of structured data in Python. If you have metrics appearing in a CSV, JSON, XML, HTML, or other supported format, either locally or via some HTTP endpoint, you can easily ingest and present those metrics in Netdata, by leveraging the Pandas collector.
This collector can be used to collect pretty much anything that can be read by Pandas, and then processed by Pandas.
The collector uses pandas to pull data and do pandas-based preprocessing, before feeding to Netdata.
This collector is supported on all platforms.
This collector supports collecting metrics from multiple instances of this integration, including remote instances.
Default Behavior
Auto-Detection
This integration doesn't support auto-detection.
Limits
The default configuration for this integration does not impose any limits on data collection.
Performance Impact
The default configuration for this integration is not expected to impose a significant performance impact on the system.
Metrics
Metrics grouped by scope.
The scope defines the instance that the metric belongs to. An instance is uniquely identified by a set of labels.
This collector is expecting one row in the final pandas DataFrame. It is that first row that will be taken
as the most recent values for each dimension on each chart using (df.to_dict(orient='records')[0]
).
See pd.to_dict()."
Per Pandas instance
These metrics refer to the entire monitored application.
This scope has no labels.
Metrics:
Metric | Dimensions | Unit |
---|
Alerts
There are no alerts configured by default for this integration.
Setup
Prerequisites
Python Requirements
This collector depends on some Python (Python 3 only) packages that can usually be installed via pip
or pip3
.
sudo pip install pandas requests
Note: If you would like to use pandas.read_sql
to query a database, you will need to install the below packages as well.
sudo pip install 'sqlalchemy<2.0' psycopg2-binary
Configuration
File
The configuration file name for this integration is python.d/pandas.conf
.
You can edit the configuration file using the edit-config
script from the
Netdata config directory.
cd /etc/netdata 2>/dev/null || cd /opt/netdata/etc/netdata
sudo ./edit-config python.d/pandas.conf
Options
There are 2 sections:
- Global variables
- One or more JOBS that can define multiple different instances to monitor.
The following options can be defined globally: priority, penalty, autodetection_retry, update_every, but can also be defined per JOB to override the global values.
Additionally, the following collapsed table contains all the options that can be configured inside a JOB definition.
Every configuration JOB starts with a job_name
value which will appear in the dashboard, unless a name
parameter is specified.
Config options
Name | Description | Default | Required |
---|---|---|---|
chart_configs | an array of chart configuration dictionaries | [] | yes |
chart_configs.name | name of the chart to be displayed in the dashboard. | None | yes |
chart_configs.title | title of the chart to be displayed in the dashboard. | None | yes |
chart_configs.family | family of the chart to be displayed in the dashboard. | None | yes |
chart_configs.context | context of the chart to be displayed in the dashboard. | None | yes |
chart_configs.type | the type of the chart to be displayed in the dashboard. | None | yes |
chart_configs.units | the units of the chart to be displayed in the dashboard. | None | yes |
chart_configs.df_steps | a series of pandas operations (one per line) that each returns a dataframe. | None | yes |
update_every | Sets the default data collection frequency. | 5 | no |
priority | Controls the order of charts at the netdata dashboard. | 60000 | no |
autodetection_retry | Sets the job re-check interval in seconds. | 0 | no |
penalty | Indicates whether to apply penalty to update_every in case of failures. | yes | no |
name | Job name. This value will overwrite the job_name value. JOBS with the same name are mutually exclusive. Only one of them will be allowed running at any time. This allows autodetection to try several alternatives and pick the one that works. | no |
Examples
Temperature API Example
example pulling some hourly temperature data, a chart for today forecast (mean,min,max) and another chart for current.
Config
temperature:
name: "temperature"
update_every: 5
chart_configs:
- name: "temperature_forecast_by_city"
title: "Temperature By City - Today Forecast"
family: "temperature.today"
context: "pandas.temperature"
type: "line"
units: "Celsius"
df_steps: >
pd.DataFrame.from_dict(
{city: requests.get(f'https://api.open-meteo.com/v1/forecast?latitude={lat}&longitude={lng}&hourly=temperature_2m').json()['hourly']['temperature_2m']
for (city,lat,lng)
in [
('dublin', 53.3441, -6.2675),
('athens', 37.9792, 23.7166),
('london', 51.5002, -0.1262),
('berlin', 52.5235, 13.4115),
('paris', 48.8567, 2.3510),
('madrid', 40.4167, -3.7033),
('new_york', 40.71, -74.01),
('los_angeles', 34.05, -118.24),
]
}
);
df.describe(); # get aggregate stats for each city;
df.transpose()[['mean', 'max', 'min']].reset_index(); # just take mean, min, max;
df.rename(columns={'index':'city'}); # some column renaming;
df.pivot(columns='city').mean().to_frame().reset_index(); # force to be one row per city;
df.rename(columns={0:'degrees'}); # some column renaming;
pd.concat([df, df['city']+'_'+df['level_0']], axis=1); # add new column combining city and summary measurement label;
df.rename(columns={0:'measurement'}); # some column renaming;
df[['measurement', 'degrees']].set_index('measurement'); # just take two columns we want;
df.sort_index(); # sort by city name;
df.transpose(); # transpose so its just one wide row;
- name: "temperature_current_by_city"
title: "Temperature By City - Current"
family: "temperature.current"
context: "pandas.temperature"
type: "line"
units: "Celsius"
df_steps: >
pd.DataFrame.from_dict(
{city: requests.get(f'https://api.open-meteo.com/v1/forecast?latitude={lat}&longitude={lng}¤t_weather=true').json()['current_weather']
for (city,lat,lng)
in [
('dublin', 53.3441, -6.2675),
('athens', 37.9792, 23.7166),
('london', 51.5002, -0.1262),
('berlin', 52.5235, 13.4115),
('paris', 48.8567, 2.3510),
('madrid', 40.4167, -3.7033),
('new_york', 40.71, -74.01),
('los_angeles', 34.05, -118.24),
]
}
);
df.transpose();
df[['temperature']];
df.transpose();
API CSV Example
example showing a read_csv from a url and some light pandas data wrangling.
Config
example_csv:
name: "example_csv"
update_every: 2
chart_configs:
- name: "london_system_cpu"
title: "London System CPU - Ratios"
family: "london_system_cpu"
context: "pandas"
type: "line"
units: "n"
df_steps: >
pd.read_csv('https://london.my-netdata.io/api/v1/data?chart=system.cpu&format=csv&after=-60', storage_options={'User-Agent': 'netdata'});
df.drop('time', axis=1);
df.mean().to_frame().transpose();
df.apply(lambda row: (row.user / row.system), axis = 1).to_frame();
df.rename(columns={0:'average_user_system_ratio'});
df*100;
API JSON Example
example showing a read_json from a url and some light pandas data wrangling.
Config
example_json:
name: "example_json"
update_every: 2
chart_configs:
- name: "london_system_net"
title: "London System Net - Total Bandwidth"
family: "london_system_net"
context: "pandas"
type: "area"
units: "kilobits/s"
df_steps: >
pd.DataFrame(requests.get('https://london.my-netdata.io/api/v1/data?chart=system.net&format=json&after=-1').json()['data'], columns=requests.get('https://london.my-netdata.io/api/v1/data?chart=system.net&format=json&after=-1').json()['labels']);
df.drop('time', axis=1);
abs(df);
df.sum(axis=1).to_frame();
df.rename(columns={0:'total_bandwidth'});
XML Example
example showing a read_xml from a url and some light pandas data wrangling.
Config
example_xml:
name: "example_xml"
update_every: 2
line_sep: "|"
chart_configs:
- name: "temperature_forcast"
title: "Temperature Forecast"
family: "temp"
context: "pandas.temp"
type: "line"
units: "celsius"
df_steps: >
pd.read_xml('http://metwdb-openaccess.ichec.ie/metno-wdb2ts/locationforecast?lat=54.7210798611;long=-8.7237392806', xpath='./product/time[1]/location/temperature', parser='etree')|
df.rename(columns={'value': 'dublin'})|
df[['dublin']]|
SQL Example
example showing a read_sql from a postgres database using sqlalchemy.
Config
sql:
name: "sql"
update_every: 5
chart_configs:
- name: "sql"
title: "SQL Example"
family: "sql.example"
context: "example"
type: "line"
units: "percent"
df_steps: >
pd.read_sql_query(
sql='\
select \
random()*100 as metric_1, \
random()*100 as metric_2 \
',
con=create_engine('postgresql://localhost/postgres?user=netdata&password=netdata')
);
Troubleshooting
Debug Mode
To troubleshoot issues with the pandas
collector, run the python.d.plugin
with the debug option enabled. The output
should give you clues as to why the collector isn't working.
Navigate to the
plugins.d
directory, usually at/usr/libexec/netdata/plugins.d/
. If that's not the case on your system, opennetdata.conf
and look for theplugins
setting under[directories]
.cd /usr/libexec/netdata/plugins.d/
Switch to the
netdata
user.sudo -u netdata -s
Run the
python.d.plugin
to debug the collector:./python.d.plugin pandas debug trace
Getting Logs
If you're encountering problems with the pandas
collector, follow these steps to retrieve logs and identify potential issues:
- Run the command specific to your system (systemd, non-systemd, or Docker container).
- Examine the output for any warnings or error messages that might indicate issues. These messages should provide clues about the root cause of the problem.
System with systemd
Use the following command to view logs generated since the last Netdata service restart:
journalctl _SYSTEMD_INVOCATION_ID="$(systemctl show --value --property=InvocationID netdata)" --namespace=netdata --grep pandas
System without systemd
Locate the collector log file, typically at /var/log/netdata/collector.log
, and use grep
to filter for collector's name:
grep pandas /var/log/netdata/collector.log
Note: This method shows logs from all restarts. Focus on the latest entries for troubleshooting current issues.
Docker Container
If your Netdata runs in a Docker container named "netdata" (replace if different), use this command:
docker logs netdata 2>&1 | grep pandas
Do you have any feedback for this page? If so, you can open a new issue on our netdata/learn repository.